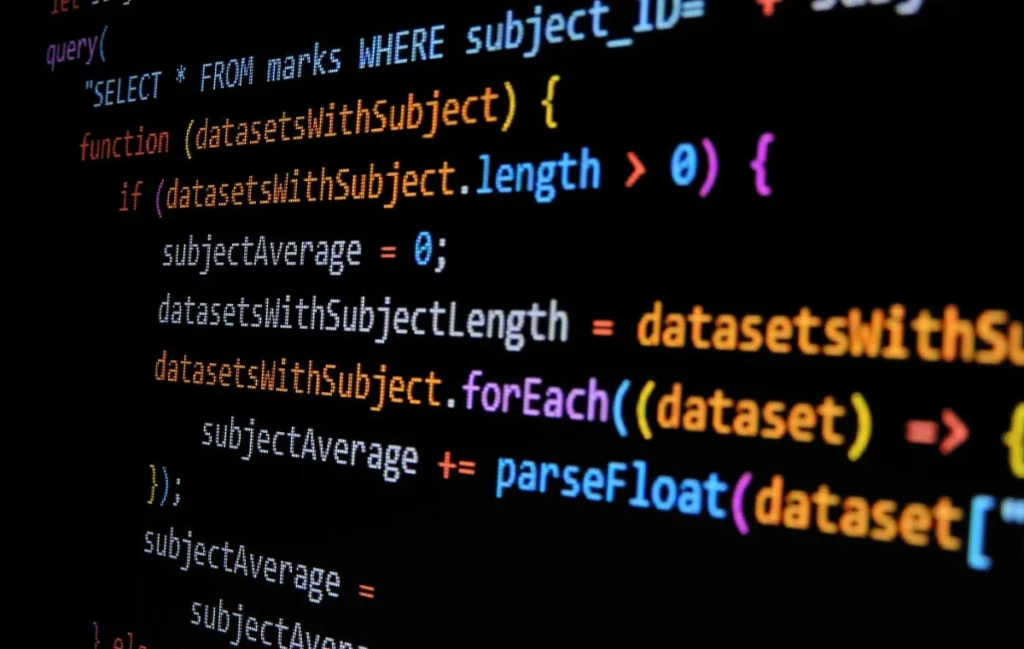
Content
Unlocking the Power of JavaScript in Modern Web Development
Introduction to JavaScript
JavaScript is a high-level programming language that has become an integral part of modern web development. Initially developed in 1995 by Brendan Eich while he was working at Netscape, JavaScript was created as a client-side scripting language to add interactivity to web pages. Over the years, it has evolved significantly, transforming from a simple scripting tool into a robust and versatile programming platform that supports both client-side and server-side applications.
Throughout its evolution, JavaScript has maintained a unique position within the web development ecosystem. It enables developers to create dynamic and interactive web applications that enhance user experience. The importance of JavaScript is further underscored by the rise of numerous frameworks and libraries, such as React, Angular, and Vue.js, which have made building complex user interfaces more streamlined and efficient. Additionally, with the introduction of Node.js, JavaScript has extended its capabilities to the server-side, allowing developers to use a single language across both client and server, thus improving development workflows and reducing the complexity of technology stacks.
Furthermore, JavaScript’s versatility is evident in its ability to interact with various web APIs, making it easier for developers to integrate third-party services and functionalities. The language itself is supported by all modern web browsers, making it universally accessible and a fundamental component of front-end development. Its importance cannot be understated, as it has established itself as the backbone of the web, empowering developers to create immersive user experiences through features such as real-time updates, animations, and responsive design.
In the context of contemporary web development, understanding JavaScript is essential for both aspiring and established developers. As the web continues to evolve, so too does the need for proficiency in JavaScript, making it a vital skill in the toolkit of any web developer.
The JavaScript Ecosystem
The JavaScript ecosystem has evolved significantly over the years, establishing a robust framework for web development. Central to this ecosystem are various libraries and frameworks that facilitate the creation of dynamic and responsive web applications. Among the most prominent frameworks are React, Angular, and Vue.js. Each of these frameworks offers unique features that cater to different development needs. For instance, React, developed by Facebook, emphasizes component-based architecture, allowing developers to build reusable UI components efficiently. Angular, on the other hand, provides a comprehensive structure for developing large-scale applications, integrating tools and functionalities that promote maintainability. Vue.js stands out for its simplicity and flexibility, making it easier for new developers to adopt and utilize in projects.
In addition to these frameworks, package management plays a critical role in the JavaScript ecosystem. Developers often utilize tools like npm (Node Package Manager) and Yarn to handle dependencies and manage project libraries effectively. These tools streamline the process of installing and maintaining packages, ensuring that projects remain organized and up to date. With npm being the default package manager for Node.js, it has garnered significant popularity, while Yarn provides an alternative that focuses on speed and efficiency in package installation and project setup.
Another key component of the JavaScript ecosystem is the runtime environment, specifically Node.js. Node.js allows JavaScript to be executed on the server side, broadening the scope of what developers can achieve with this versatile language. It provides an event-driven architecture capable of handling concurrent requests, making it suitable for developing scalable applications. The synergy among JavaScript frameworks, package management tools, and runtime environments contributes to a thriving ecosystem that continues to evolve, driving modern web development practices forward.
Key Features of JavaScript
JavaScript offers a multitude of features that empower developers to create highly interactive and dynamic web applications. One of the standout characteristics of JavaScript is its status as a first-class function. This means that functions can be treated as variables, allowing them to be assigned to variables, passed as arguments, or returned from other functions. A simple example would be the ability to pass a function as an argument to the Array method map
:
Asynchronous programming in JavaScript has seen significant advancements with the introduction of Promises and the async/await syntax. Promises provide a mechanism to handle asynchronous operations effectively, enabling developers to write cleaner code without getting caught in callback hell. For instance:
With the async/await syntax, developers can write asynchronous code that reads more like synchronous code, making it easier to follow and maintain:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error:', error); }}
Prototypal inheritance is another key feature that distinguishes JavaScript from other programming languages. It enables objects to inherit properties and methods from other objects, facilitating a more flexible and dynamic object-oriented approach. This can be illustrated as follows:
function Animal(name) { this.name = name;}Animal.prototype.speak = function() { console.log(`${this.name} makes a noise.`);};const dog = new Animal('Dog');dog.speak(); // Outputs: Dog makes a noise.
Finally, the modern ES6+ syntax enhances JavaScript’s expressiveness and simplification through features like arrow functions, template literals, and destructuring assignment. This improved syntax not only makes the code more readable but also reduces the likelihood of errors, thereby streamlining the development process.
JavaScript in Frontend Development
JavaScript plays a pivotal role in frontend development, primarily due to its capability to create responsive and interactive user interfaces. As a client-side scripting language, it enables developers to enhance the functionality of web applications, ensuring an engaging user experience. One of the key components of this integration is the Document Object Model (DOM), which provides a structured representation of the document. Through the DOM, JavaScript allows developers to manipulate HTML and CSS, dynamically altering the content and style of web pages in real-time.
By utilizing JavaScript, developers can access and modify various elements of the DOM, making it easier to manage user interactions. For instance, JavaScript can respond to user inputs such as clicks, keystrokes, or form submissions. This event handling is essential for creating dynamic interactions, enhancing the overall usability of a web application. Furthermore, the ability to add animations and transitions improves the visual appeal, encouraging users to engage more deeply with the content.
Another crucial aspect of modern frontend development is the use of Asynchronous JavaScript and XML (AJAX). This technique allows a web page to communicate with a server in the background, retrieving data without forcing a full page reload. By fetching data asynchronously, developers can build smoother and faster user experiences, which is critical in today’s fast-paced online environment. AJAX facilitates the implementation of rich features such as live search results, real-time notifications, and auto-updating content, all of which are integral to maintaining user engagement.
In conclusion, JavaScript is indispensable in frontend development. Its ability to manipulate the DOM, handle events, and implement AJAX provides developers the tools necessary to create responsive, interactive, and dynamic web applications. As web standards continue to evolve, the demand for skilled JavaScript developers remains high, emphasizing the language’s significance in modern web development.
JavaScript for Backend Development
JavaScript has evolved significantly from its origins as a client-side scripting language. Today, it plays a pivotal role in backend development, allowing developers to build comprehensive applications using a single programming language. This transition has primarily been facilitated by Node.js, a runtime environment that enables JavaScript to run server-side.
Node.js is renowned for its event-driven, non-blocking I/O model that makes it efficient and suitable for data-intensive real-time applications. With its ability to handle numerous simultaneous connections, Node.js allows for the development of scalable network applications. By leveraging JavaScript on the backend, developers achieve full-stack capabilities, simplifying the development process and unifying the codebase across the client and server.
To streamline the creation of server-side applications, Express.js, a minimal and flexible Node.js web application framework, is commonly employed. Express simplifies the process of routing and middleware management, enhancing the performance and scalability of JavaScript-based applications. It has become the standard framework for building RESTful APIs and web applications, providing a robust layer on top of Node.js that improves developer productivity.
Furthermore, integrating databases is a vital aspect of backend development. JavaScript allows for seamless connections to various databases, most notably MongoDB, a widely-used NoSQL database. Using MongoDB’s native JavaScript API, developers can efficiently store and retrieve data in a format that aligns with JavaScript objects, facilitating smoother data manipulation and retrieval.
Incorporating these technologies—Node.js, Express.js, and MongoDB—unlocks tremendous potential for building robust and scalable server-side applications. As JavaScript continues to dominate both client-side and backend development, its position as a versatile language in modern web development is firmly established.
Best Practices for JavaScript Development
In the realm of modern web development, adhering to best practices in JavaScript coding is essential for creating clean, maintainable, and efficient code. To begin with, establishing coding standards is crucial. These standards promote consistency throughout the codebase, making it easier for developers to read and understand each other’s work. Utilizing tools like ESLint can help enforce these conventions by identifying stylistic errors and potential code issues early in the development process.
Documentation plays a vital role in JavaScript development as well. Clear and comprehensive documentation serves as a reference for both current and future developers, elucidating the purpose of various functions and the overall architecture of the application. It can also assist in onboarding new team members, allowing them to understand the codebase more rapidly. Tools such as JSDoc can be invaluable in generating structured documentation straight from the code, enhancing clarity and accessibility.
Another essential principle in JavaScript development is modular programming. By breaking down a codebase into smaller, manageable modules, developers can enhance code reusability and improve maintainability. This approach allows teams to work on different modules simultaneously, thus accelerating the development process. Utilizing frameworks such as React, Angular, or Vue.js further facilitates modular development, as they encourage component-based architecture.
Moreover, the significance of unit testing cannot be overstated. Writing unit tests ensures that individual components of the application function as intended, which ultimately contributes to a more stable and reliable product. Employing testing frameworks like Jest or Mocha helps automate this process, allowing developers to quickly identify and resolve issues before deployment.
By adhering to these best practices—coding standards, proper documentation, modular programming, and unit testing—developers can significantly enhance the quality and effectiveness of their JavaScript projects. Furthermore, utilizing supportive tools and frameworks will continue to streamline the development process, allowing teams to harness the full potential of JavaScript in modern web development.
The Role of JavaScript in Responsive Design
JavaScript plays a pivotal role in enhancing responsive web design, serving as a powerful tool alongside HTML and CSS to create dynamic and engaging user experiences. One of the primary advantages of JavaScript in responsive design is its ability to facilitate dynamic content adjustment. For instance, JavaScript can be used to alter text, images, and layouts based on the user’s screen size or orientation, which allows for a more tailored viewing experience. This kind of interactivity is increasingly crucial as more users access the web on a range of devices including smartphones, tablets, and desktops.
Additionally, JavaScript can enhance responsive image handling by allowing developers to implement features such as lazy loading and image resizing based on the user’s device. This responsiveness ensures that images are loaded appropriately for different screen resolutions, helping to reduce load times and improve overall performance. By leveraging JavaScript, developers can also implement sliders and galleries that adjust their content appropriately as the viewport changes, further enhancing usability and engagement.
Another key aspect is the integration of media queries within JavaScript. While CSS primarily handles media queries for style adaptations, JavaScript can manipulate certain elements dynamically based on the media query thresholds. This capability enables the execution of more complex behaviors when specific conditions are met, creating a seamless transition of functionalities across varying devices. Furthermore, popular frameworks such as Bootstrap have incorporated JavaScript components that are inherently responsive, allowing for easier implementation and a unified design approach.
In conclusion, JavaScript is a vital component in modern responsive web design. Its ability to dynamically adjust content and implement interactive elements enhances the overall user experience across devices. By effectively using JavaScript in conjunction with CSS frameworks like Bootstrap, developers can ensure their websites are not only visually appealing but also functionally robust for all users, regardless of the device they choose.
JavaScript and Web APIs
JavaScript plays a crucial role in modern web development by enabling seamless interaction with various web APIs. Web APIs allow developers to leverage built-in browser capabilities, thereby enhancing user experience and functionality in applications. One prominent example is the Fetch API, which simplifies the process of making network requests. With the Fetch API, developers can easily retrieve resources from the network, such as JSON data from a server, and process it within their applications. This capability is particularly important for single-page applications (SPAs), where content needs to be dynamically loaded without refreshing the browser.
Another important API that JavaScript interacts with is the Geolocation API. This API provides a way for web applications to access the user’s geographical location. Drawing on the device’s GPS, Wi-Fi, or cellular network data, the Geolocation API enables features such as location-based services, allowing developers to create applications that offer customized experiences based on where the user is located. For instance, weather applications can display local weather conditions, while mapping services can provide directions tailored to the user’s current whereabouts.
Furthermore, WebSockets represent a groundbreaking advancement in real-time communication. This protocol allows persistent connections between the client and server, facilitating bi-directional messaging. JavaScript’s integration with WebSockets enables developers to build applications that require immediate data exchange, such as chat applications or live notifications. For instance, in online gaming platforms, the ability to receive real-time updates significantly enhances user engagement and interactivity.
Overall, JavaScript’s interaction with web APIs is a cornerstone of modern web development. It empowers developers to create dynamic, responsive applications that can efficiently handle data, cater to user location, and maintain real-time communication, all of which are essential in delivering a comprehensive web experience.
The Future of JavaScript
As web development continues to evolve, JavaScript stands out as one of the most prominent programming languages. Current trends indicate that JavaScript is poised for a fascinating future, with significant advancements that will shape how developers create web applications. One of the primary areas of growth is in the development of new frameworks and libraries that simplify complex processes and enhance user experience. Frameworks such as React, Angular, and Vue.js have gained immense popularity, prompting continuous updates and new features that cater to a diverse range of development needs.
In addition, the recent announcement of features in ECMAScript, the standardized version of JavaScript, showcases a commitment to keeping the language relevant. Features such as optional chaining, nullish coalescing, and top-level await are mere examples of how JavaScript is becoming more developer-friendly, allowing for cleaner code and enhanced functionality. Looking forward, it is anticipated that ECMAScript will introduce even more innovative capabilities. The focus will likely shift towards improving performance, security, and scalability to meet the demands of modern web applications.
Furthermore, the rise of TypeScript—an extension of JavaScript that incorporates static typing—suggests a trend towards more robust, large-scale applications. As teams aim to increase code reliability and maintainability, TypeScript’s acceptance among developers is likely to grow. Another exciting development lies in the integration of JavaScript with emerging technologies such as Artificial Intelligence (AI) and Machine Learning (ML). This integration will enable developers to create more interactive, intelligent web applications that cater to user preferences and behavior.
In conclusion, the future of JavaScript is bright and filled with exciting possibilities. As the language continues to evolve, developers can expect a dynamic landscape that embraces innovation and meets the changing demands of web users. Emphasizing trends in framework development, feature enhancements, and the convergence with cutting-edge technologies will ultimately propel JavaScript into new realms of digital creation.
Follow us on our Facebook page: Facebook
Click here to see our Work which consists of modern and functional websites that make a difference.